Find the number of lowercase characters in a String using Python 3:
In this python tutorial, we will learn how to find the total number of lowercase characters in a string. The user will enter one string, our program will count the total lowercase characters in that string and print out the result. The string can contain a mix of characters, numbers and any other special characters. Even for an empty string, this program will work. For checking if a character is lowercase or not, we will use one inbuilt method. Python provides one method to verify if a character is uppercase or lowercase easily. We will use that one. Before going into details how the program looks like, let me quickly explain you the algorithm we are going to use.
Algorithm :
-
First of all, take the string as an input from the user. Store it in a separate variable.
-
Initialize one variable counter as ‘0’. This counter will store the total count of all lowercase characters. We will iterate through the string characters one by one and we will increment the counter by one if any lowercase character is found.
-
Iterate through the string character by character. We will use one loop to iterate through the string.
-
Check for each character if it is lowercase or uppercase. We will use one inbuilt method to check if the current character is lowercase or uppercase.
-
If it is a lowercase character, increment the counter by one. Else, move to the next character. The counter variable will hold the total count of lowercase characters at the end of the loop.
-
Finally, check if the counter is more than ‘0’ or not. If it is more than ‘0’, print the number. If it is less than ‘0’, print that ‘No lowercase Character is found.’
Python 3 Program :
input_string = input("Enter a string : ")
count = 0
for c in input_string:
if(c.islower()):
count = count + 1
if(count == 0):
print("No Lower case character is found in the string.")
else :
print("Total no of lower case character : ",count)
You can also download this program from here.
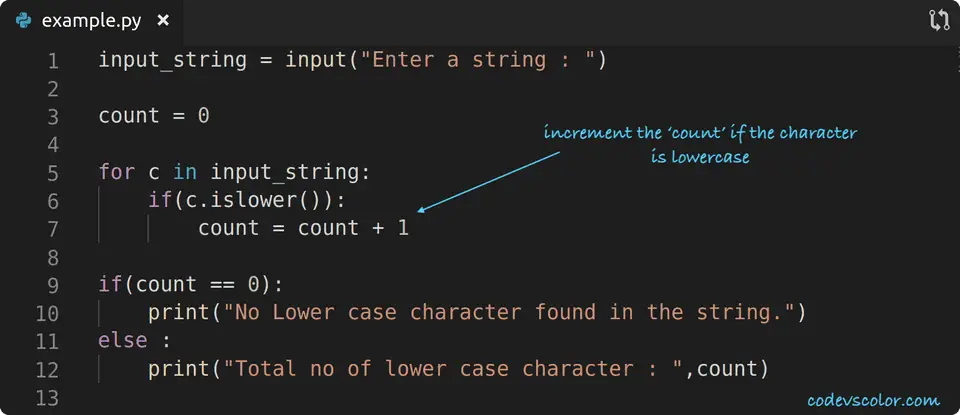
Explanation of the program:
-
First, take the string input from user using ‘input()’. input() method reads the user input value as a string.
-
save the input in a variable ‘input_string’. ‘input_string’ is holding the string we read in the previous step.
-
use a ‘for’ loop to scan all the characters of the string one by one. In python, we can easily iterate through the string characters as you can see in the example above.
-
Check if a character is lowercase using ‘.islower()’ method inside the for loop. This will check if a character is lowercase on each iteration of the for loop, or it will verify if a character is lowercase or not for all characters of the given string. This method returns True if the character is lowercase. Else, it returns False.
-
If it is ‘true’, increment the ‘count’ value. It should be initialized with value ‘0’. We will increment the ‘count’ variable by one if any lowercase character is found.
-
After the ‘for’ loop is completed, check if ‘count’ is ‘0’ or more than ‘0’. If ‘0’, that means no lower case character is found. Print the value of ‘count’ if it is more than ‘0’. This ‘count’ variable will hold the total lowercase character count.
Example program :
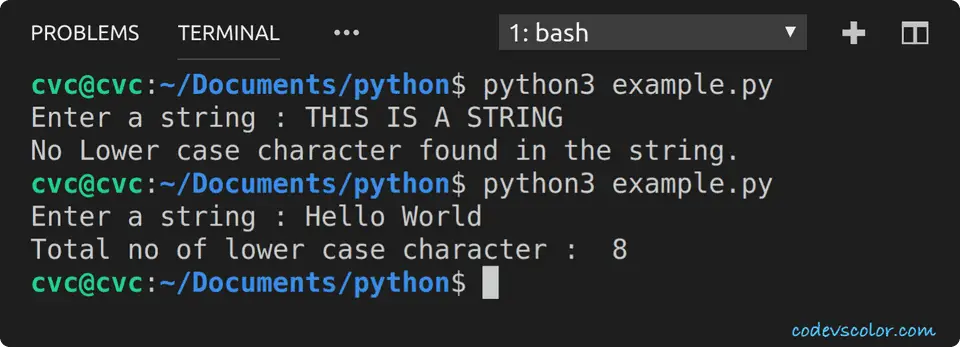