Python 3 program to count the number of blank spaces in a file :
In this tutorial, we will learn how to find the total number of blank spaces in a text file. This program will teach you basic file operations in python. You will learn how to iterate the lines of a text file and how to read each word and each character for each line.
You will also learn how to check if a character is a blank space or not. The program is easier than you think.
The following algorithm we are going to use in this example :
Algorithm :
- Store the file path in a variable. This is the path of the text file we are going to read. In this example, we are using one dummy file path.
Before running the program, change this path to the file path you are going to test on your system. You can simply drag and drop one file on a terminal session to get the file path.
-
Create one variable to store the count of blank spaces and initialize it as ‘0’. We will keep updating this variable whenever we find any blank space in the file.
-
Open the file and read the lines one by one.
-
For each line, read all words one by one.
-
For each word, read all characters one by one. Actually, we are reading all the characters of the file one by one. But to do that, we need all these three steps.
-
Check for each character if it is a space or not. Python provides one inbuilt method to check if a character is a space or not. We will use that one.
-
If the character is a space, increment the count variable by one. The initial value of the count variable is 0. If any space is found, it will become 1, for the next space, it will be 2 etc. At the end of the program, this variable will hold the total count of space in the file.
-
Finally, print out the value of count or the count of blank spaces to the user.
Python 3 program :
file_path = "input.txt"
space_count = 0
with open(file_path, 'r') as f:
for line in f:
split_words = line.split()
for word in split_words:
for char in word:
if(char.isspace):
space_count = space_count + 1
print("Total blank space found : ", space_count)
You can also download this program from here.
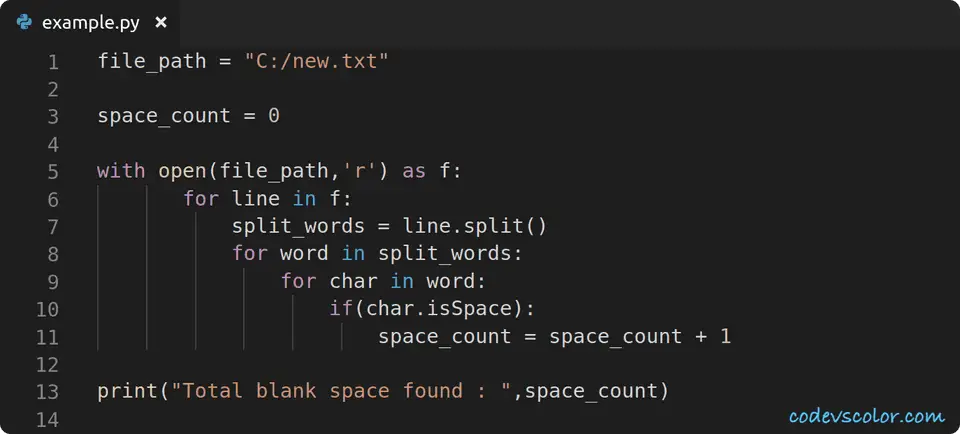
-
In this example, we are reading the file in read mode using ‘open(file_path,’r’)’ method. open() method is used to open a file. It takes two parameters: the first one is the file path we want to open and the second one is the mode of operation. We are only reading the contents of the file, so ‘r’ is used to denote that this is only a read operation. Don’t forget to change the value of file_path before running the program.
-
Using a ‘for’ loop, we are reading all lines one by one. For each line, ‘.split()’ method is used to get all the words.
-
It will split the line into a list of words.
-
Now, use one more ‘for’ loop and iterate through all words.
-
For each word, use one ‘for’ loop and iterate through each character of that word.
-
Check for each character – if it is space or not using the ‘.isspace’ property.
-
If it is a space, increment the counter.
-
Finally, print out the counter.
Similar tutorials :
- List all the files in a Zip file using Python 3
- Python program to count the number of words in a file
- Python tutorial to remove duplicate lines from a text file
- Python program to count the total number of lines in a file
- Python program to rename a directory or file
- Python program to delete all files with specific extension in a folder