Python 3 program to check if a string is pangram or not:
In this tutorial, we will learn how to check if a string is pangram or not using python 3.
A pangram string contains every letter of a given alphabet. For example, ‘the quick brown fox jumps over the lazy dog’ is a pangram in English. But ‘abcdefghij’ is not.
Similarly, ”The five boxing wizards jump quickly.” and ”Pack my box with five dozen liquor jugs.” are also pangram.
A perfect pangram contains every letter of the alphabet only once.
Our program will ask the user to enter one string. It will verify if it is a pangram or not and print out the result.
Let me quickly explain the algorithm we are using in this problem :
Algorithm :
-
Ask the user to enter a string. Read it, and store it in a variable.
-
We can solve this problem in different ways. In this example, we will learn how to solve it by using a Set. Set is used to hold unique items in Python, i.e. a Set can’t hold any duplicate items.
First of all, we will convert all characters of the string to lowercase and put them in a set.
If more than two same characters are found in the string, only one will be included in the set. e.g. for the string ‘hello world’, the set will be {‘h’, ‘e’, ‘l’, ‘o’, ‘w’, ‘r’, ‘d’}.
Now, create one new set by taking all alphabets in the set and find out the size difference between them.
If the size is 0, the string is a pangram. Else, it is not.
Python Program :
def check_pangram(arg):
if len(set('abcdefghijklmnopqrstuvwxyz') - set(arg.lower())) == 0 :
return True
return False
user_str = input("Enter a string to check for pangram : ")
if(check_pangram(user_str)):
print("It is a pangram string")
else:
print("Not a pangram string")
You can also download this program from here
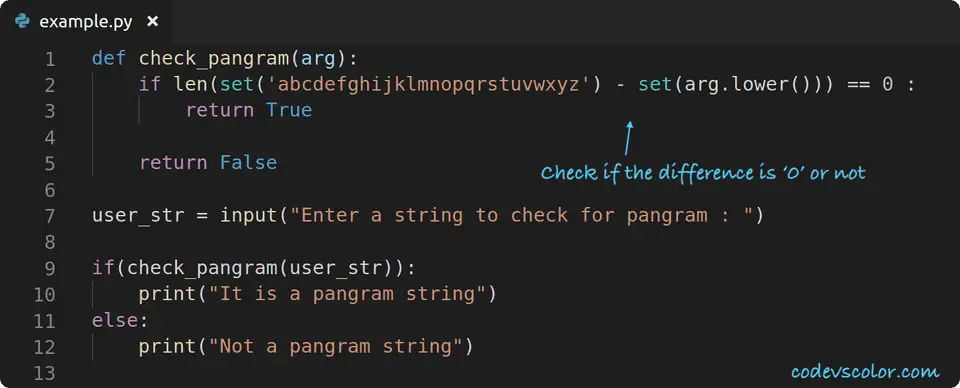
Output :
Explanation :
-
We are defining one separate method ‘check_pangram’ to check if a string is a pangram or not.
-
‘check_pangram’ method takes one string as its argument and checks if it is pangram or not. The main idea of this method is that ‘set’ cannot contain duplicate elements. So, if we create a set of all characters of the input string, it will filter out the characters. Before creating a set, we are converting all characters to lowercase using ‘lower’.
-
For example, for the string ‘Hello World‘, the set will contain ‘e,l,h,o,w,r,d’. We already have one set with all characters set(‘abcdefghijklmnopqrstuvwxyz’). So, if we subtract the new set from this set, it will contain the elements that exist in the set(‘abcdefghijklmnopqrstuvwxyz’) and don’t exist in the new set.
We are checking the count of the final set. If the count is ‘0’, means the second set contains all alphabets. In this case, return ‘True’. If the count is not ‘0’, return ‘False’.
Conclusion :
In this tutorial, we have learned how to find out if a string is pangram string or not using python. You can use the same concept to create a pangram checker program in any other programming language. Try to implement the above program and drop one comment below if you have any queries.
Similar tutorials :
- Python swap case of each character of a string
- Python example program to split a string at linebreak using splitlines
- Python string center method explanation with example
- Python program to calculate total vowels in a string
- Python program to check if two strings are an anagram or not
- Python program to count the words and characters in a string