Introduction :
Toast messages are used to show one simple message to the user for a short interval of time. Unlike any popup messages, toast doesn’t block any UI activities. If you want to show a simple feedback message, toast is the right way to do it.
React native provides an API to show a toast message easily. We can also customize the toast like display time, position etc. In this tutorial, we will learn how this API works and its features.
Create one React native application :
We are using the npx to create this project. Run the following command to create one react native project with the name Example :
npx react-native init Example
It will create a project with name Example.
Adding a Toast to the react-native project :
Note that Toast is a native feature of Android. It doesn’t work on iOS. Make sure to test the application properly on an iPhone if you are planning to release it on AppStore.
Open the App.js file in your project. This is the main file to draw the main layout of the start screen view of a react native project. Add the below code to this file :
/**
* Sample React Native App
* https://github.com/facebook/react-native
*
* @format
* @flow
*/
import React, { Component } from "react";
import { Platform, StyleSheet, Button, View } from "react-native";
import { ToastAndroid } from "react-native";
export default class App extends Component<Props> {
showToast = () => {
ToastAndroid.showWithGravityAndOffset(
"Hello World !!",
ToastAndroid.LONG,
ToastAndroid.BOTTOM,
25,
50
);
};
constructor(props) {
super(props);
this.state = {
visible: false,
};
}
render() {
return (
<View style={styles.container}>
<Button title="Click Me" onPress={this.showToast} />
</View>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: "center",
alignItems: "center",
backgroundColor: "#F5FCFF",
},
});
Run the application on an Android device using npx react-native run-android command. It will create one screen like below :
If you click on the button, it will show you the Toast as below.
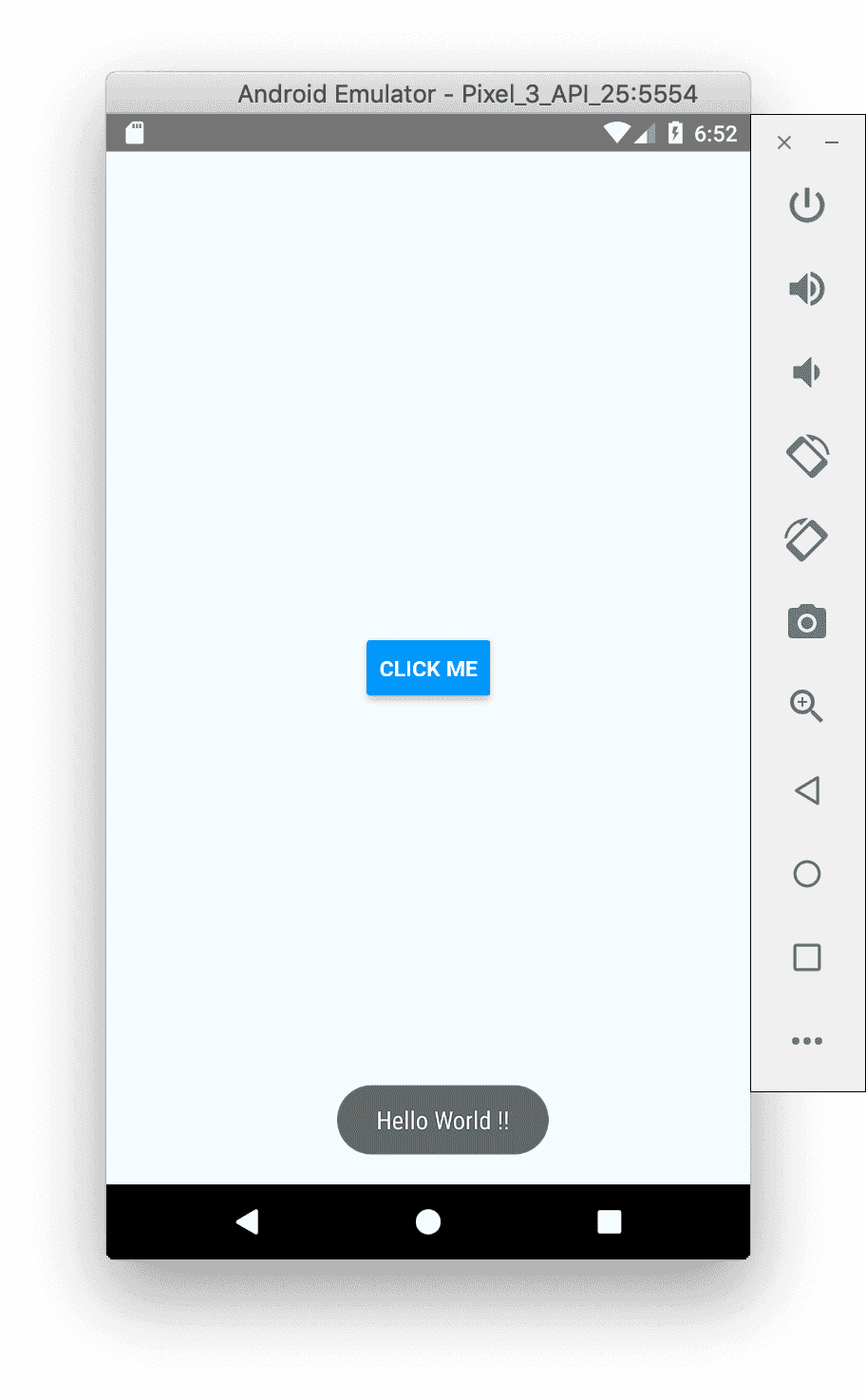
Toast methods:
Following methods are available for the ToastAndroid API :
1. static show(message, duration) :
Use it to show a toast with a message for a specific duration.
2. static showWithGravity(message, duration, gravity) :
With this method, you can add gravity to the toast. Three types of gravity are supported: top, bottom and centre.
3. static showWithGravityAndOffset(message, duration, gravity, xOffset, yOffset) :
You can also add x and y offset along with gravity to the toast. In the above example, we are using this method.
Duration :
Two different durations are available :
SHORT: ToastAndroid.SHORT;
LONG: ToastAndroid.LONG;
Gravity :
Three gravity values are available :
TOP: ToastAndroid.TOP;
BOTTOM: ToastAndroid.BOTTOM;
CENTER: ToastAndroid.CENTER;
Conclusion :
You can always add gravity and offset to a toast. The offset values are translated to pixels. So, it may look different on different phones. Try to go through the program we have seen above and drop one comment below if you have any queries.
You might also like:
- React Navigation part 6: How to set and change the header title
- React navigation tutorial 7: How to add header and header text color, font etc.
- React navigation tutorial 8: How to add one image as the header title
- How to add buttons on header in React navigation
- How to use one WebView in react native
- How to create blur view in React Native
- How to create horizontal scrollbar with views in React native